728x90
반응형
목차
- 개발 환경 설정
- Spring Boot 프로젝트 생성
- H2 데이터베이스 설정
- 엔터티(Entity)
- H2 웹 테스트
1. 개발 환경 설정
필수 소프트웨어
- JDK 17 이상 : Spring Boot 3.x는 최소 JDK 17을 요구함.
- IDE : Eclipse 추천
- Maven: 프로젝트 빌드 도구
Download the Latest Java LTS Free
Subscribe to Java SE and get the most comprehensive Java support available, with 24/7 global access to the experts.
www.oracle.com
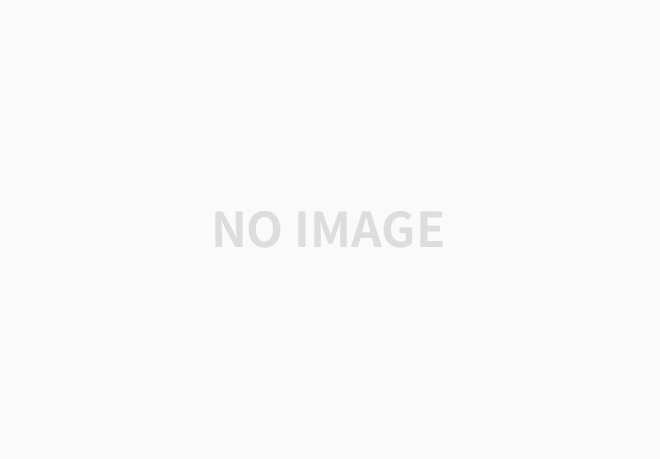
2. Spring Boot 프로젝트 생성
Spring Initializr를 사용하여 간단히 프로젝트를 생성할 수 있습니다.
https://start.spring.io
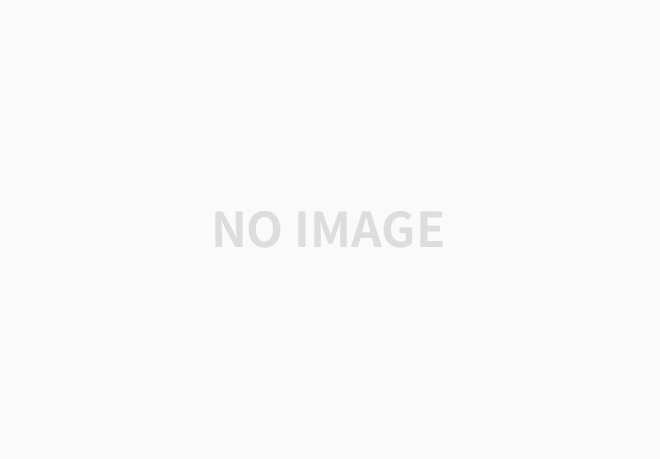
프로젝트를 다운로드하고 IDE에서 열기.
다운 받은 demo.zip파일을 압축풀고
IDE에서 import + Existing maven Projects 으로 불러옵니다
3. H2 데이터베이스 설정
1) application.properties 설정
Spring Boot에서 H2 데이터베이스는 기본적으로 메모리 기반 데이터베이스를 지원하기에 이를 활성화합니다.
application.properties(주의!! 띄어쓰기, 들여쓰기, 빈칸 있으면 안됩니다.)
logging.level.root=DEBUG
spring.jpa.show-sql=true
spring.h2.console.enabled=true
spring.datasource.url=jdbc:h2:mem:testdb
spring.h2.console.path=/h2-console
주요 설정:
- spring.datasource.url=jdbc:h2:mem:testdb : 메모리 기반 데이터베이스(testdb라는 이름 사용).
- spring.h2.console.enabled=true : H2 웹 콘솔 활성화.
- spring.h2.console.path=/h2-console : H2 웹 콘솔 경로 설정.
4. 엔터티(Entity) 생성
1) 엔터티 클래스 생성
JPA는 엔티티 클래스(Demo)를 참조해 데이터베이스 테이블을 생성합니다.
package com.example.cardatabase.domain;
import jakarta.persistence.Column;
import jakarta.persistence.Entity;
//import jakarta.persistence.FetchType;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
//import jakarta.persistence.JoinColumn;
//import jakarta.persistence.ManyToOne;
@Entity
public class Demo {
@Id
@GeneratedValue(strategy=GenerationType.AUTO)
private long id;
private String brand, model, color, registerNumber;
@Column(name="`year`")
private int year;
private int price;
public Demo() {}
public Demo(String brand, String model, String color,
String registerNumber, int year, int price) {
super();
this.brand = brand;
this.model = model;
this.color = color;
this.registerNumber = registerNumber;
this.year = year;
this.price = price;
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public String getRegisterNumber() {
return registerNumber;
}
public void setRegisterNumber(String registerNumber) {
this.registerNumber = registerNumber;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
}
5. H2 웹 테스트
Spring Boot 애플리케이션을 실행한 뒤, H2 콘솔에 접속해 웹에서 확인 가능.
- 애플리케이션 실행: ./mvnw spring-boot:run
- 브라우저에서 http://localhost:8080/h2-console 로 접속.
- 다음 정보 입력:
- JDBC URL: jdbc:h2:mem:testdb
- Username: sa
- Password: (비워 둠)
'IT개발 > Spring Boot3' 카테고리의 다른 글
[Spring Boot3] 의존성 주입(Dependency Injection) 이해하기(이걸로 끝) (0) | 2025.03.20 |
---|---|
[Spring Boot3] JSP를 사용하지 않는 이유 & JPA, JSON 개념(간단) (0) | 2025.03.20 |
[Spring Boot3]@RestController, @JsonIgnore, @RequestBody 정의 및 예시 (0) | 2024.11.25 |
[Spring Boot3]로그인과 쿠키, 세션, 인터셉터 (0) | 2024.11.24 |
[Spring5] 커맨드 객체 검증(Validate(), @Valid, Bean Validation) (0) | 2024.11.24 |